
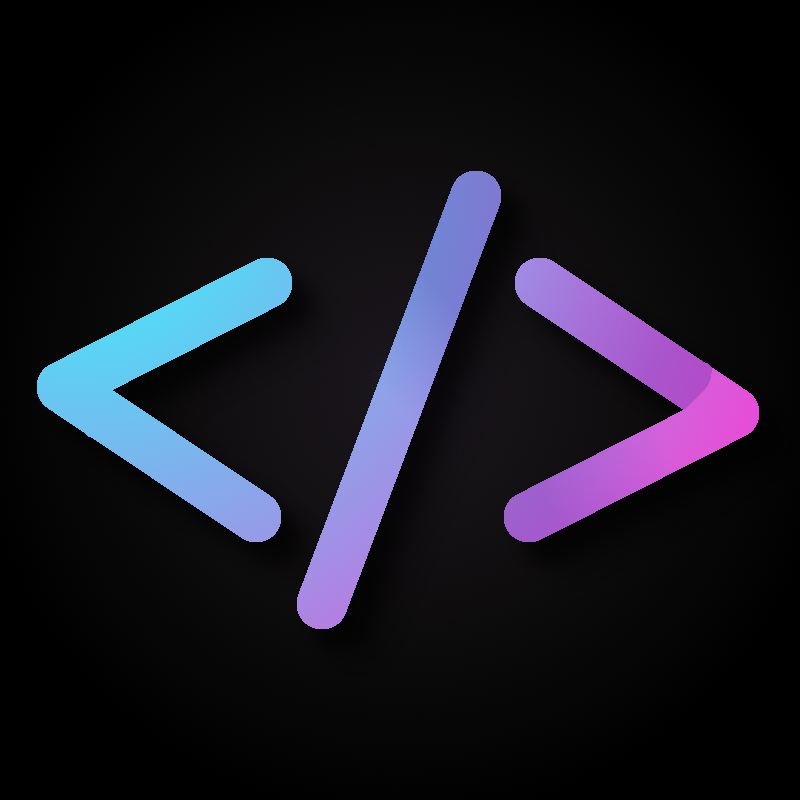
Hmmm… That’s true, my rough litmus test is “can you explain what this thing does in fairly precise language without having to add a bunch of qualifiers for different cases?”
If you meet that bar the function is probably fine/doesn’t need broken up further.
That said, I don’t particularly care how many functions I have to jump through or what their line count is because I can verify “did the function do the thing it says it’s supposed to do?” after it’s called in a debugger. If it did, then I know my bug isn’t there. If it didn’t, I know where to look.
Just like with commits, I’d rather have more small commits to feed through git bisect than a few larger commits because it makes identifying where/when a contract/test case/invariant was violated much more straight forward.
It sounds like just from how you describe things Swift is using fibers instead of real OS threads(?)
What are these things/what is this comparing?