
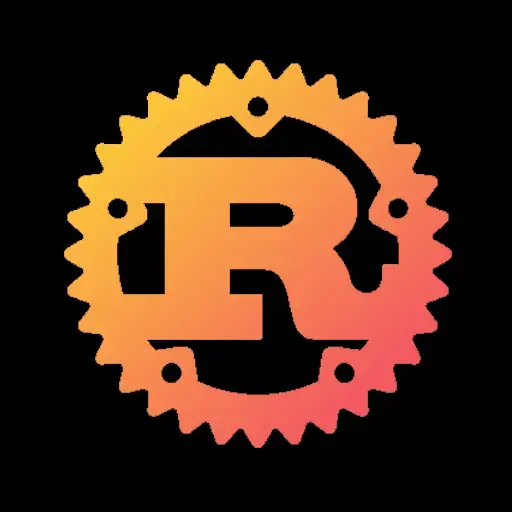
Disclaimer that I have no experience with writing compilers myself, but conceptually I don’t see any obvious reason that someone couldn’t create a JIT compiler for Rust so that it can be treated like a scripting language and do rapid iteration…
Disclaimer that I have no experience with writing compilers myself, but conceptually I don’t see any obvious reason that someone couldn’t create a JIT compiler for Rust so that it can be treated like a scripting language and do rapid iteration…
I’m not familiar enough with what you’re trying to do to offer any specific advice. I’ve spent very little time with writing dockerfiles, and have never needed to set up a Rust toolchain in a dockerfile.
I think the first step is figuring out if nightly is really needed. If there aren’t any nightly features needed then the latest stable toolchain should work fine, and worrying about what version of the toolchain to use is a red herring.
Nightly is for language features of Rust that are still being tested or experimented with. It should only be used by developers who are eager for the latest bleeding edge capabilities and are willing to adapt if those capabilities change or get dropped entirely. Or you might use nightly if you’re a good citizen and testing out the experimental capabilities so that you can give feedback on them.
A later version of nightly could potentially change or drop the features of an earlier version of nightly in ways that are not backwards compatible. That’s why you might have to specify which version of nightly you need (potentially an older version), if you’re building something that depended on nightly features.
This is exactly the use case that slotmap is meant for. I highly recommend using the library rather than reinventing the concept.
In Deep Space 9 there’s an episode called “Trials and Tribble-ations” where a number of the crew from DS9 go back in time and find themselves in the TOS episode “Trouble with Tribbles”. The producers literally overlayed the DS9 cast onto TOS footage, and shot some new clips in the same setting, so canonically the DS9 crew was present for the events of the TOS episode (… at least as canonically as you can get when time travel is involved).
Warf and O’Brien were two of the DS9 crew involved in that episode, and they also happen to be on the Enterprise when Scotty is found trapped in a pattern buffer.
If you put an underscore as the first character in the variable name, that tells the compiler that the variable may go unused and you’re okay with that. E.g.
let mut _last = &mut first;
When it comes to certifying your own software, the evidence is the key thing needed by the certifying bodies.
If I’m someone who wants to get my Rust software safety certified, it’s not enough for me to tell the certifier “I compiled my software with this compiler from a project called Ferocene and they have a blog post saying that they’re safety certified. Give me a certification please.”
Instead you need to show the certifier all the evidence that went into the safety case that achieved certification for Ferocene and then make a case that the evidence is relevant to your own safety case. This evidence from Ferocene is what you pay them for.
And you’ve pretty much made it over the tipping point into being a Rustacean when you embrace the fact that it is a good thing that Rust doesn’t let you do things the way you’re familiar with from other languages because those familiar ways were always the source of immense suffering without you even realizing it.
Mirror universe Janeway would use the caretaker technology to conquer the Delta Quadrant and become the Borg queen without even being assimilated.
Using visual studio code this only happens if the library has thorough type annotation. While that’s becoming more popular, it’s not enforced at the language level so lots of libraries have enormous gaps in the autocomplete.
It only took me ~2 weeks of playing with Rust before it became my scripting language of choice over Python (which I had been using casually for ~5 years by that point).
The initial setup for Rust can be whipped up with $ cargo init
. You’re right that there’s more setup boilerplate because of the mandatory Cargo.toml
and directory structure, but cargo init
will provide all that in a snap.
As for the domain specific boilerplate, I actually find that Rust is better at that than Python in almost all cases. I feel that Rust’s clap
is much simpler, more reliable, and less boilerplate than Python’s argparse. Python might win in cases where there’s a very mature domain specific package that you need which isn’t available in the Rust ecosystem, but that’s becoming rare as crates.io grows.
And then when it comes to the actual “scripting”, very often my IDE’s intellisense can practically fill in the Rust code for me. One keystroke per word and it knows what function I want, or I can quickly scroll through the recommendations until I find what I’m looking for. Meanwhile with Python I always have to consult docs to find any API that isn’t part of the basic standard library. As a result I’ll often get the scripting done faster in Rust than in Python.
It does absolutely take some time to reach that point, though. Most programmers will definitely feel significant discomfort with Rust initially, but that’s just because you need to deprogram your brain from the bad habits that other languages encourage. There’s a tipping point in that deprogramming where all the other languages start to feel uncomfortable because you know it won’t let you write as good quality of code as Rust would.
Considering most JIT compilers for JavaScript are written in C++, I can’t conceive of a reason you couldn’t implement one in Rust.
Is part of your requirement that
unsafe
doesn’t get used anywhere in the dependency tree? If so you’d have to take away most of the Ruststd
library since many implementations in there have small strategic uses ofunsafe
under the hood.In my entire software engineering career, which spans embedded systems to CAD applications, I’ve never encountered a case where
GOTO
is actually needed (but maybe some places where it can be used as a dirty shortcut to save you some lines of code).As for arbitrary function pointers, if those function pointers are written in Rust then they’ll come with all the safety assurances afforded to Rust code. I suppose if you’re worried about the danger of running ussr-code with
unsafe
in it, you could probably have your JIT refuse to compile theunsafe
keyword specifically.